11: Numpy - Slicing and Indexing#
import numpy as np
Indexing and Slicing#
The operation is the same as for lists
A = np.array([[1, 2, 3], [4, 5, 6]])
print(A)
[[1 2 3]
[4 5 6]]
# To access row 0, column 1
A[0, 1]
2
# To select blocks in row (0-1) column (0-1)
A[0:2, 0:2]
array([[1, 2],
[4, 5]])
A[0:2, 0:2] = 10
print(A)
[[10 10 3]
[10 10 6]]
Boolean Indexing#
A = np.array([[1, 2, 3], [4, 5, 6]])
print(A<5) # Boolean mask
print(A[A < 5]) # subset filtered by the boolean mask
A[A<5] = 4 # convert the selected values.
print(A)
[[ True True True]
[ True False False]]
[1 2 3 4]
[[4 4 4]
[4 5 6]]
Exercises and Solutions#
Exercise 1#
Fill the 4 blocks of the middle with “1’s
B = np.zeros((4, 4))
B
array([[0., 0., 0., 0.],
[0., 0., 0., 0.],
[0., 0., 0., 0.],
[0., 0., 0., 0.]])
# SOLUTION
B[1:3 , 1:3] = 1
B
array([[0., 0., 0., 0.],
[0., 1., 1., 0.],
[0., 1., 1., 0.],
[0., 0., 0., 0.]])
Exercise 2#
Fill in the table of “1” (every other row, every other column)
C = np.zeros((5, 5))
C
array([[0., 0., 0., 0., 0.],
[0., 0., 0., 0., 0.],
[0., 0., 0., 0., 0.],
[0., 0., 0., 0., 0.],
[0., 0., 0., 0., 0.]])
# SOLUTION
C[::2, ::2] = 1
C
array([[1., 0., 1., 0., 1.],
[0., 0., 0., 0., 0.],
[1., 0., 1., 0., 1.],
[0., 0., 0., 0., 0.],
[1., 0., 1., 0., 1.]])
Exercise 3#
On the image below, perform a slicing to keep only half of the image (in its center) and replace all pixels > 150 by pixels = 255
from scipy import misc
import matplotlib.pyplot as plt
face = misc.face(gray=True)
plt.imshow(face, cmap=plt.cm.gray)
plt.show()
face.shape
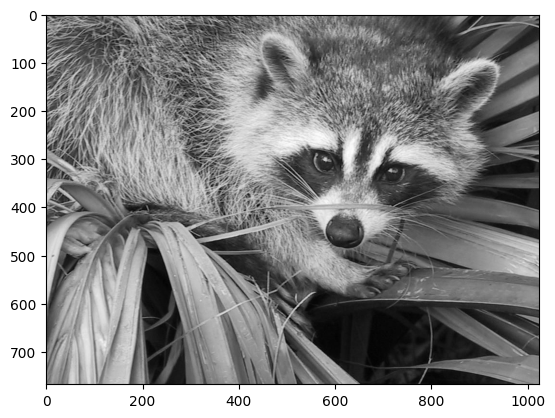
(768, 1024)
SOLUTION#
Show code cell content
x, y = face.shape
zoom_face = face[x//4 : -x//4, y //4: -y//4] # resize by dividing each dimension by 4 (integer division)
zoom_face[zoom_face>150] = 255 # boolean indexing
plt.imshow(zoom_face, cmap=plt.cm.gray)
plt.show()
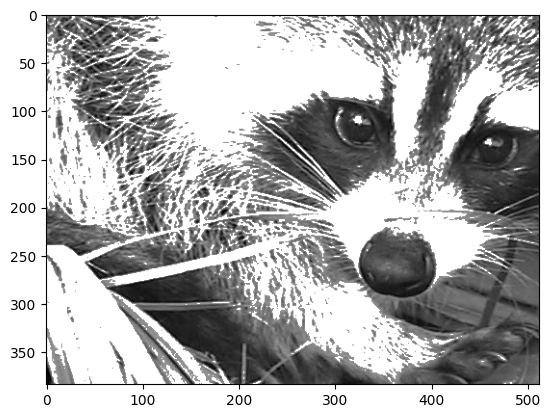